What is an enumerator in C# 2024?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Isabella Phillips
Studied at the University of Buenos Aires, Lives in Buenos Aires, Argentina.
As a domain expert in programming languages, particularly C#, I am well-versed in the intricacies of its features and constructs. Let's delve into the concept of an enumerator in C#.
An enumerator, commonly referred to as an "enum" in C#, is a value type that represents a set of named integer constants. It provides a more readable and maintainable way to work with groups of related constants. Enums are declared using the `enum` keyword and are a powerful tool for enhancing code clarity and reducing the likelihood of errors associated with using magic numbers (unnamed constants).
### Declaration of Enums
To declare an enum, you start with the `enum` keyword followed by the name of the enumeration. Here’s a simple example:
```csharp
public enum DaysOfWeek
{
Sunday,
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday
}
```
In this example, `DaysOfWeek` is an enum that contains a set of named constants representing the days of the week.
### Underlying Type
Enums in C# are based on an underlying integral type, which by default is `int`. However, you can specify a different underlying type such as `byte`, `sbyte`, `short`, `long`, `uint`, `ulong`, etc. Here’s an example with an underlying type of `int`:
```csharp
public enum FileAccess : int
{
Read = 1,
Write = 2,
Execute = 4
}
```
### Using Enums
Enums can be used in switch statements, if statements, and anywhere else you would use an integer. They provide a clear and expressive way to handle a set of predefined values.
```csharp
public void DisplayDayOfWeek(DaysOfWeek day)
{
switch (day)
{
case DaysOfWeek.Monday:
Console.WriteLine("Monday is the first day of the week.");
break;
// Other cases...
}
}
```
### Enum Members and Values
Each member of an enum is associated with a value. If you don’t explicitly assign a value to an enum member, it automatically gets the next integer value, starting from zero for the first member. However, you can manually assign values to control the exact integer
An enumerator, commonly referred to as an "enum" in C#, is a value type that represents a set of named integer constants. It provides a more readable and maintainable way to work with groups of related constants. Enums are declared using the `enum` keyword and are a powerful tool for enhancing code clarity and reducing the likelihood of errors associated with using magic numbers (unnamed constants).
### Declaration of Enums
To declare an enum, you start with the `enum` keyword followed by the name of the enumeration. Here’s a simple example:
```csharp
public enum DaysOfWeek
{
Sunday,
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday
}
```
In this example, `DaysOfWeek` is an enum that contains a set of named constants representing the days of the week.
### Underlying Type
Enums in C# are based on an underlying integral type, which by default is `int`. However, you can specify a different underlying type such as `byte`, `sbyte`, `short`, `long`, `uint`, `ulong`, etc. Here’s an example with an underlying type of `int`:
```csharp
public enum FileAccess : int
{
Read = 1,
Write = 2,
Execute = 4
}
```
### Using Enums
Enums can be used in switch statements, if statements, and anywhere else you would use an integer. They provide a clear and expressive way to handle a set of predefined values.
```csharp
public void DisplayDayOfWeek(DaysOfWeek day)
{
switch (day)
{
case DaysOfWeek.Monday:
Console.WriteLine("Monday is the first day of the week.");
break;
// Other cases...
}
}
```
### Enum Members and Values
Each member of an enum is associated with a value. If you don’t explicitly assign a value to an enum member, it automatically gets the next integer value, starting from zero for the first member. However, you can manually assign values to control the exact integer
2024-07-19 01:22:19
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at Google, Lives in London. Graduated from Stanford University with a degree in Computer Science.
The enum keyword is used to declare an enumeration, a distinct type that consists of a set of named constants called the enumerator list. + Usually it is best to define an enum directly within a namespace so that all classes in the namespace can access it with equal convenience.
2023-05-07 06:12:59
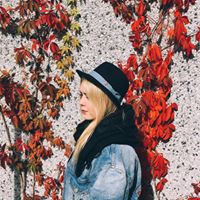
Scarlett Price
QuesHub.com delivers expert answers and knowledge to you.
The enum keyword is used to declare an enumeration, a distinct type that consists of a set of named constants called the enumerator list. + Usually it is best to define an enum directly within a namespace so that all classes in the namespace can access it with equal convenience.