What is the purpose of a function prototype 2024?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
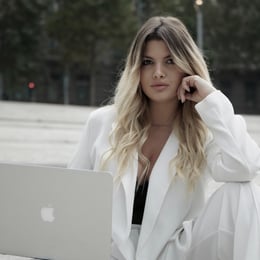
Zoe Wilson
Studied at the University of Melbourne, Lives in Melbourne, Australia.
Hello! I'm a seasoned software engineer with over a decade of experience in designing and implementing software solutions. I've worked with a multitude of programming languages over the years and have a deep understanding of the intricacies of software development.
Let's dive into the concept of function prototypes and why they are a fundamental aspect of many programming languages.
## The Essence of Function Prototypes
In the realm of programming, a function prototype serves as a declaration of a function to the compiler *before* its actual definition. It acts like a blueprint, providing the compiler with essential information about the function, even if the complete implementation of the function isn't yet known.
Think of it this way: Imagine you're building a house. You wouldn't start constructing walls without a blueprint that outlines the structure, dimensions, and placement of each room, would you? Similarly, function prototypes provide the compiler with a blueprint of how the function will be used.
## Key Information Conveyed by a Function Prototype
A function prototype typically includes the following pieces of information:
1. Return Type: This specifies the type of data the function will return after it completes its task. For instance, if a function is designed to calculate the area of a rectangle and return the result, the return type might be `int` (for integer) or `double` (for a decimal value).
2. Function Name: Just like every house needs an address, every function needs a unique identifier. This is the name by which the function will be called and invoked within the program.
3. Parameter List: Parameters are like the ingredients a function needs to work with. The parameter list specifies the type and order of data that the function expects to receive as input. For example, a function calculating the area of a rectangle would likely accept two parameters: the length and width, both probably of type `int` or `double`.
## The Crucial Role of Function Prototypes
But why are these prototypes so important? Here's where their significance shines through:
1. Early Error Detection: One of the most critical roles of prototypes is to help the compiler catch errors early in the development process. By declaring the function's interface upfront, the compiler can cross-check every time the function is called to ensure that:
* The correct number of arguments are being passed.
* The data types of the arguments match what the function expects.
* The return value is being used correctly based on its declared type.
This early detection prevents subtle bugs from creeping into your code and saves you hours of debugging later on.
2. Code Organization and Readability: Imagine reading a book where all the chapters are jumbled up. That's what code can feel like without function prototypes. Prototypes promote a top-down approach to programming, allowing you to define the high-level structure of your program first without getting bogged down in implementation details. This makes your code more organized, readable, and easier to maintain.
3. Facilitating Modular Programming: Prototypes are essential for modular programming, a software design technique where programs are broken down into smaller, independent modules. By declaring function prototypes in header files, you can separate the interface of a module from its implementation. This allows different parts of a program, or even different programs, to use the same functions without needing to know the specifics of how those functions are implemented.
## Illustrative Example
Let's solidify our understanding with a simple example in C++:
```c++
#include <iostream>
// Function prototype
int calculateArea(int length, int width);
int main() {
int roomLength = 10;
int roomWidth = 5;
// Calling the function
int area = calculateArea(roomLength, roomWidth);
std::cout << "The area of the room is: " << area << std::endl;
return 0;
}
// Function definition
int calculateArea(int length, int width) {
return length * width;
}
```
In this example, the line `int calculateArea(int length, int width);` is the function prototype. It tells the compiler that a function named `calculateArea` exists, takes two integers as input, and returns an integer value. The actual implementation of the function comes later.
Let's dive into the concept of function prototypes and why they are a fundamental aspect of many programming languages.
## The Essence of Function Prototypes
In the realm of programming, a function prototype serves as a declaration of a function to the compiler *before* its actual definition. It acts like a blueprint, providing the compiler with essential information about the function, even if the complete implementation of the function isn't yet known.
Think of it this way: Imagine you're building a house. You wouldn't start constructing walls without a blueprint that outlines the structure, dimensions, and placement of each room, would you? Similarly, function prototypes provide the compiler with a blueprint of how the function will be used.
## Key Information Conveyed by a Function Prototype
A function prototype typically includes the following pieces of information:
1. Return Type: This specifies the type of data the function will return after it completes its task. For instance, if a function is designed to calculate the area of a rectangle and return the result, the return type might be `int` (for integer) or `double` (for a decimal value).
2. Function Name: Just like every house needs an address, every function needs a unique identifier. This is the name by which the function will be called and invoked within the program.
3. Parameter List: Parameters are like the ingredients a function needs to work with. The parameter list specifies the type and order of data that the function expects to receive as input. For example, a function calculating the area of a rectangle would likely accept two parameters: the length and width, both probably of type `int` or `double`.
## The Crucial Role of Function Prototypes
But why are these prototypes so important? Here's where their significance shines through:
1. Early Error Detection: One of the most critical roles of prototypes is to help the compiler catch errors early in the development process. By declaring the function's interface upfront, the compiler can cross-check every time the function is called to ensure that:
* The correct number of arguments are being passed.
* The data types of the arguments match what the function expects.
* The return value is being used correctly based on its declared type.
This early detection prevents subtle bugs from creeping into your code and saves you hours of debugging later on.
2. Code Organization and Readability: Imagine reading a book where all the chapters are jumbled up. That's what code can feel like without function prototypes. Prototypes promote a top-down approach to programming, allowing you to define the high-level structure of your program first without getting bogged down in implementation details. This makes your code more organized, readable, and easier to maintain.
3. Facilitating Modular Programming: Prototypes are essential for modular programming, a software design technique where programs are broken down into smaller, independent modules. By declaring function prototypes in header files, you can separate the interface of a module from its implementation. This allows different parts of a program, or even different programs, to use the same functions without needing to know the specifics of how those functions are implemented.
## Illustrative Example
Let's solidify our understanding with a simple example in C++:
```c++
#include <iostream>
// Function prototype
int calculateArea(int length, int width);
int main() {
int roomLength = 10;
int roomWidth = 5;
// Calling the function
int area = calculateArea(roomLength, roomWidth);
std::cout << "The area of the room is: " << area << std::endl;
return 0;
}
// Function definition
int calculateArea(int length, int width) {
return length * width;
}
```
In this example, the line `int calculateArea(int length, int width);` is the function prototype. It tells the compiler that a function named `calculateArea` exists, takes two integers as input, and returns an integer value. The actual implementation of the function comes later.
2024-06-21 09:51:52
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at the International Seabed Authority, Lives in Kingston, Jamaica.
In computer programming, a function prototype or function interface is a declaration of a function that specifies the function's name and type signature (arity, data types of parameters, and return type), but omits the function body.
2023-04-20 05:22:49
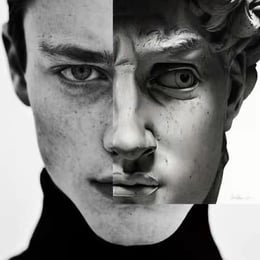
Lucas Brown
QuesHub.com delivers expert answers and knowledge to you.
In computer programming, a function prototype or function interface is a declaration of a function that specifies the function's name and type signature (arity, data types of parameters, and return type), but omits the function body.