What is the Spring MVC framework 2024?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Isabella Bailey
Studied at the University of Toronto, Lives in Toronto, Canada.
Hi there! I'm a software engineer with over a decade of experience in building web applications, and I've worked extensively with Spring MVC. I'd be happy to break down what it is and how it works.
## Diving Deep into Spring MVC: A Robust Framework for Web Applications
Spring MVC, short for Spring Model-View-Controller, is a robust and widely used framework for building enterprise-level Java web applications. It's built on top of the core principles of the Spring Framework, inheriting its powerful dependency injection and aspect-oriented programming capabilities, while adding specialized features tailored for web development.
At its core, Spring MVC helps you structure your application by adhering to the Model-View-Controller (MVC) architectural pattern, a widely adopted approach to designing software applications, particularly those involving user interfaces. Let's understand each component of this pattern in the context of Spring MVC:
1. Model:
* Role: This component represents the data of your application and the business logic that operates on it. Think of it as the heart of your application, responsible for managing and manipulating the information that your application handles.
* In Spring MVC: You'll often find this represented by Java classes, often annotated with Spring annotations like `@Component`, `@Service`, or `@Repository`, depending on their specific role. These classes encapsulate data structures, interact with databases, and implement the core logic of your application.
2. View:
* Role: This component is responsible for presenting the model data to the user in a visually engaging way. Essentially, it's what the user sees and interacts with in their web browser.
* In Spring MVC: Spring provides excellent support for various view technologies, including JSP (JavaServer Pages), Thymeleaf, FreeMarker, and Velocity. These technologies offer different ways to render dynamic content within HTML pages, allowing you to display data retrieved from the model and create interactive user interfaces.
3. Controller:
* Role: This acts as the intermediary between the Model and the View. It receives requests from the user, typically through interactions with the view, processes these requests often by interacting with the model, and then selects the appropriate view to display the results back to the user.
* In Spring MVC: You define controllers using Java classes annotated with `@Controller`. Within these controllers, you write methods (mapped to specific URLs using annotations like `@RequestMapping`) to handle incoming requests, interact with the model, and ultimately return a response to the user, often by specifying which view should be rendered.
## How Spring MVC Works: A Request's Journey
Understanding how a request flows through a Spring MVC application is crucial to grasping its elegance and efficiency. Here's a step-by-step breakdown:
1. Request Arrival: When a user interacts with your web application – say, by clicking a link or submitting a form – their web browser sends an HTTP request to the server where your application is running.
2. DispatcherServlet Takes Charge: The heart of Spring MVC is the `DispatcherServlet`. This central component intercepts all incoming requests, acting like a traffic controller.
3. Handler Mapping: The `DispatcherServlet` consults a mechanism called a *Handler Mapping*. This component's job is to find the appropriate controller (and the specific method within that controller) that's designed to handle the incoming request, based on the request URL and other factors.
4. Controller Execution: The identified controller method is executed. This method might interact with the model – fetching data from a database, performing calculations, or updating information.
5. Model Updates: After processing the request, the controller typically populates a `ModelAndView` object. This object holds the data (the "model") that needs to be displayed to the user and optionally specifies the name of the view to render.
6. View Rendering: The `DispatcherServlet` consults a *View Resolver* to map the logical view name (provided by the controller) to an actual view implementation (like a JSP or a Thymeleaf template).
7.
View Preparation: The selected view template is then processed. Any dynamic content placeholders within the template are populated with the actual data from the model, effectively merging the view with the model.
8.
Response Generation: Finally, the fully rendered view, now containing the requested data and presented in a user-friendly format, is sent back as an HTTP response to the user's browser. The user sees the result of their interaction.
## Key Advantages of Spring MVC
* Clear Separation of Concerns: The MVC pattern inherent in Spring MVC enforces a clean division between data, logic, and presentation. This makes your code more organized, easier to maintain,...
## Diving Deep into Spring MVC: A Robust Framework for Web Applications
Spring MVC, short for Spring Model-View-Controller, is a robust and widely used framework for building enterprise-level Java web applications. It's built on top of the core principles of the Spring Framework, inheriting its powerful dependency injection and aspect-oriented programming capabilities, while adding specialized features tailored for web development.
At its core, Spring MVC helps you structure your application by adhering to the Model-View-Controller (MVC) architectural pattern, a widely adopted approach to designing software applications, particularly those involving user interfaces. Let's understand each component of this pattern in the context of Spring MVC:
1. Model:
* Role: This component represents the data of your application and the business logic that operates on it. Think of it as the heart of your application, responsible for managing and manipulating the information that your application handles.
* In Spring MVC: You'll often find this represented by Java classes, often annotated with Spring annotations like `@Component`, `@Service`, or `@Repository`, depending on their specific role. These classes encapsulate data structures, interact with databases, and implement the core logic of your application.
2. View:
* Role: This component is responsible for presenting the model data to the user in a visually engaging way. Essentially, it's what the user sees and interacts with in their web browser.
* In Spring MVC: Spring provides excellent support for various view technologies, including JSP (JavaServer Pages), Thymeleaf, FreeMarker, and Velocity. These technologies offer different ways to render dynamic content within HTML pages, allowing you to display data retrieved from the model and create interactive user interfaces.
3. Controller:
* Role: This acts as the intermediary between the Model and the View. It receives requests from the user, typically through interactions with the view, processes these requests often by interacting with the model, and then selects the appropriate view to display the results back to the user.
* In Spring MVC: You define controllers using Java classes annotated with `@Controller`. Within these controllers, you write methods (mapped to specific URLs using annotations like `@RequestMapping`) to handle incoming requests, interact with the model, and ultimately return a response to the user, often by specifying which view should be rendered.
## How Spring MVC Works: A Request's Journey
Understanding how a request flows through a Spring MVC application is crucial to grasping its elegance and efficiency. Here's a step-by-step breakdown:
1. Request Arrival: When a user interacts with your web application – say, by clicking a link or submitting a form – their web browser sends an HTTP request to the server where your application is running.
2. DispatcherServlet Takes Charge: The heart of Spring MVC is the `DispatcherServlet`. This central component intercepts all incoming requests, acting like a traffic controller.
3. Handler Mapping: The `DispatcherServlet` consults a mechanism called a *Handler Mapping*. This component's job is to find the appropriate controller (and the specific method within that controller) that's designed to handle the incoming request, based on the request URL and other factors.
4. Controller Execution: The identified controller method is executed. This method might interact with the model – fetching data from a database, performing calculations, or updating information.
5. Model Updates: After processing the request, the controller typically populates a `ModelAndView` object. This object holds the data (the "model") that needs to be displayed to the user and optionally specifies the name of the view to render.
6. View Rendering: The `DispatcherServlet` consults a *View Resolver* to map the logical view name (provided by the controller) to an actual view implementation (like a JSP or a Thymeleaf template).
7.
View Preparation: The selected view template is then processed. Any dynamic content placeholders within the template are populated with the actual data from the model, effectively merging the view with the model.
8.
Response Generation: Finally, the fully rendered view, now containing the requested data and presented in a user-friendly format, is sent back as an HTTP response to the user's browser. The user sees the result of their interaction.
## Key Advantages of Spring MVC
* Clear Separation of Concerns: The MVC pattern inherent in Spring MVC enforces a clean division between data, logic, and presentation. This makes your code more organized, easier to maintain,...
2024-06-21 09:54:32
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Amsterdam, Lives in Amsterdam, Netherlands.
The Spring Web model-view-controller (MVC) framework is designed around a DispatcherServlet that dispatches requests to handlers, with configurable handler mappings, view resolution, locale and theme resolution as well as support for uploading files.
2023-04-22 05:22:51
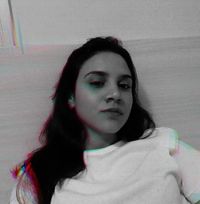
Isabella Carter
QuesHub.com delivers expert answers and knowledge to you.
The Spring Web model-view-controller (MVC) framework is designed around a DispatcherServlet that dispatches requests to handlers, with configurable handler mappings, view resolution, locale and theme resolution as well as support for uploading files.